Using the standard Timer function is a good way to get started with writing C++ code. Here are some of the things you can do with this function: Read a 16-bit timer, detect when a timer has overflowed, and delete a timer.
Read a 16-bit timer
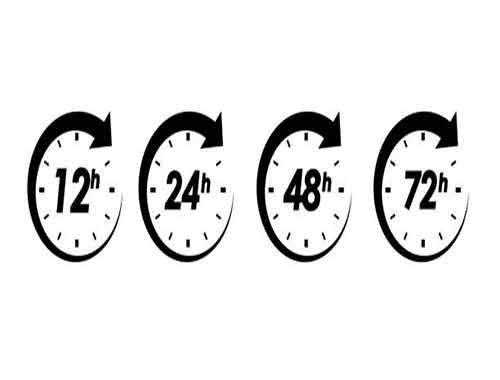
Getting a 16-bit timer to work can be a challenge. Thankfully, AVRs have a special trick to prevent corruption of data. The first step is to disable interrupts during a 16-bit read. The next step is to write to the temp register which contains the value of the high byte. This is done in the same clock cycle as the low byte is written.
This enables AVRs to access 16-bit registers via an 8-bit data bus. The AVR is well suited to this task, as its designers knew that corruption was an issue with 16-bit hardware.
The AVR also makes use of a single 8-bit register to temporarily store the high byte of the 16-bit timer. This enables the timer to be accessed in its native form. The 16-bit timer can be found in a variety of microcontrollers, ranging from a handful of PICs to some NXP Cortex M3 parts. These devices can count upwards of 4 billion input clocks, Check out the post right here.
The Timer1 module, which is included with most PIC(r) MCU devices, is a 16-bit timer/counter that has several useful built-in features. For example, it can be used as a time base for a Capture/Compare function. The module can also be used to calculate the opacity of a lightbulb. The module can even be used to resize photos and videos.
Detect when a timer has overflowed
Detecting when a timer has overflowed when using a standard timer function can be done with several methods. Some of these methods involve checking the result of a calculation after it has been performed. Others involve evaluating the resulting value. Regardless of the method used, detecting an overflow can be critical to the reliability and security of a program.
A common cause of program errors is unanticipated arithmetic overflow. This occurs when the ideal result of an integer operation is closer to minus infinity than the output type’s representable value. This can result in unintended behavior and may also compromise the program’s security.
Many computers have dedicated processor flags for overflow conditions. Some languages also provide overflow exceptions, which are a safe way to detect the occurrence of overflow.
When the CPU detects an overflow, it will set the overflow flag bit in the TCON register. The CPU then executes special code to deal with the overflow. In some cases, this will cause the counter to reset back to zero.
In other cases, the counter will be incremented. If the value is high, it will overflow and cause the timer to reset. This can lead to an arbitrary code execution and potentially lead to a buffer overflow. In addition, the overflow results will be stored as the least significant representable digits.
Delete a timer
Using a timer as a decorator can be an elegant way to modify the behaviour of a function. This can improve readability of code and make functions simpler to understand.
A timer is a time-triggered script routine that runs regularly after an interval of time. This is useful for testing whether an application is running or not. The timer can be disabled or enabled, and its text can be customized.
A timer can also be used as a context manager. Like a decorator, this function can be inserted before or after a block of code. The context stores additional information about the trigger.
The function is similar to the Python context manager. The main advantage of using a timer as a decorator is that it has less boilerplate code. It is also easier to monitor the runtime of complete functions.
Another advantage of the decorator is that it can be safely deleted from the Timer class. However, you need to ensure that you do not remove the xTimer parameter of the function. If you do, then the code will still be present.
A timer can also be used to measure the interval between alerts. This is a useful alternative to delays, which can take a long time to trigger. The interval between alerts should be greater than the amount of time you want to wait for your function to execute.
Summary: